In this year’s first issue of my irregular Django quick tips series, lets look at the builtin tools available for managing access control.
The framework offers a comprehensive authentication and authorization system that is able to handle the common requirements of most websites without even needing any external library.
Most of the time, simple websites only make use of the “authentication” features, such as registration, login and logout. On more complex systems only authenticating the users is not enough, since different users or even groups of users will have access to distinct sets of features and data records.
This is when the “authorization” / access control features become handy. As you will see they are very simple to use as soon as you understand the implementation and concepts behind them. Today I’m gonna focus on how to use these permissions on the Admin, perhaps in a future post I can address the usage of permissions on other situations. In any case Django has excellent documentation, so a quick visit to this page will tell you what you need to know.
Under the hood
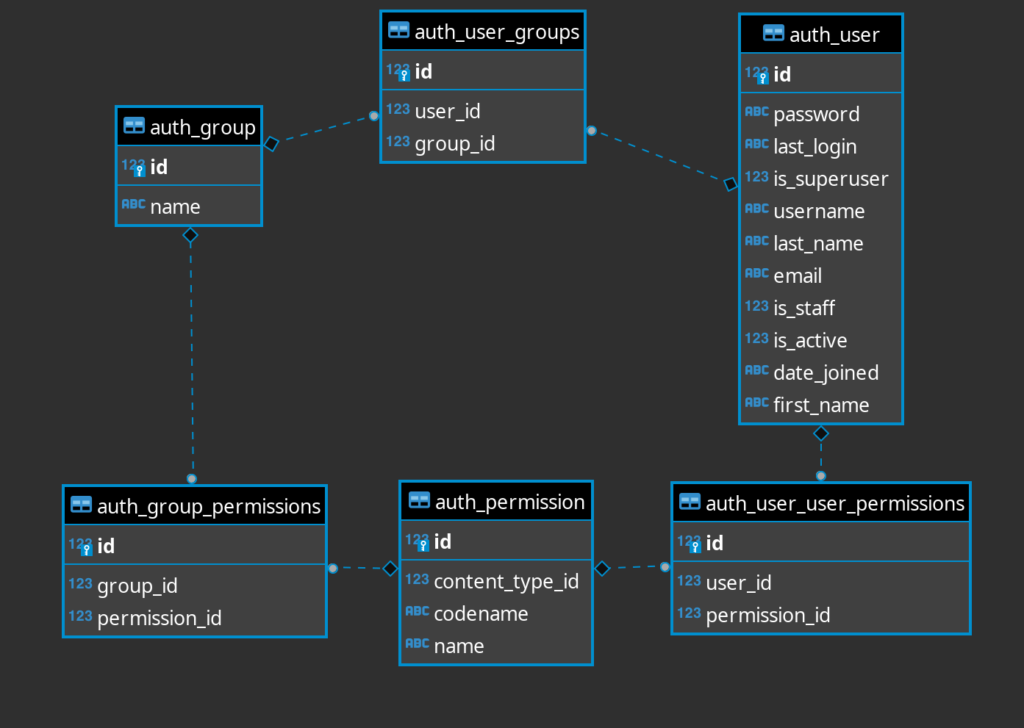
The above picture is a quick illustration of how this feature is laid out in the database. So a User can belong to multiple groups and have multiple permissions, each Group can also have multiple permissions. So a user has a given permission if it is directly associated with him or or if it is associated with a group the user belongs to.
When a new model is added 4 permissions are created for that particular model, later if we need more we can manually add them. Those permissions are <app>.add_<model>
, <app>.view_<model>
, <app>.update_<model>
and <app>.delete_<model>
.
For demonstration purposes I will start with these to show how the admin behaves and then show how to implement an action that’s only executed if the user has the right permission.
The scenario
Lets image we have a “store” with some items being sold and it also has a blog to announce new products and promotions. Here’s what the admin looks like for the “superuser”:
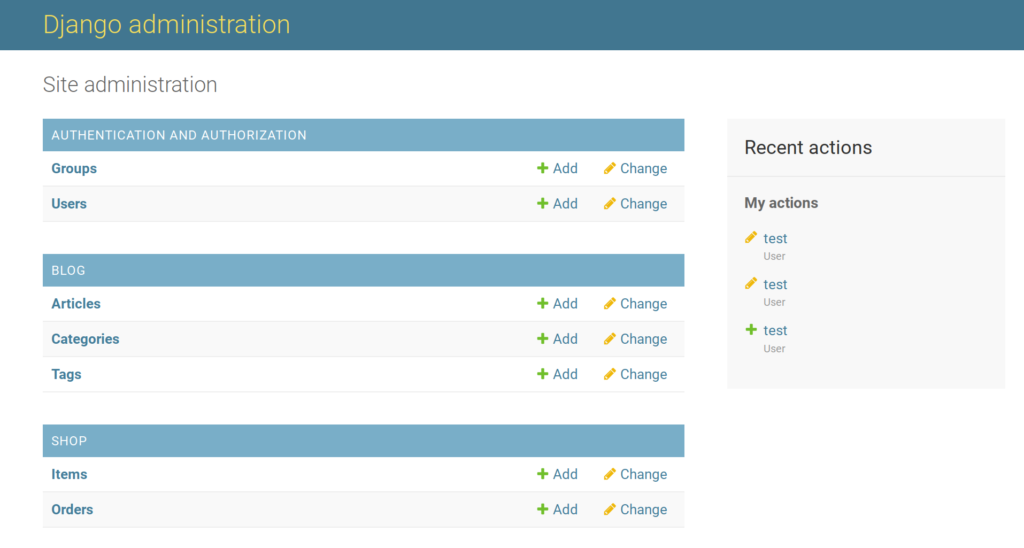
We have several models for the described functionality and on the right you can see that I added a test user. At the start, this test user is just marked as regular “staff” (is_staff=True
), without any permissions. For him the admin looks like this:
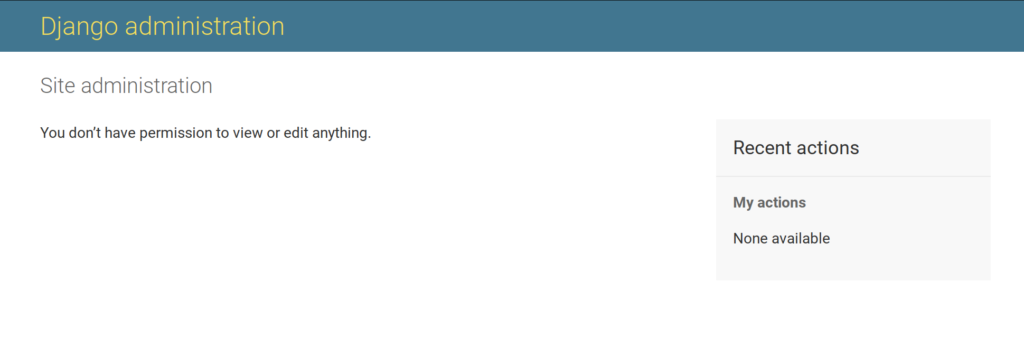
After logging in, he can’t do anything. The store manager needs the test user to be able to view and edit articles on their blog. Since we expect in the future that multiple users will be able to do this, instead of assigning these permissions directly, lets create a group called “editors” and assign those permissions to that group.
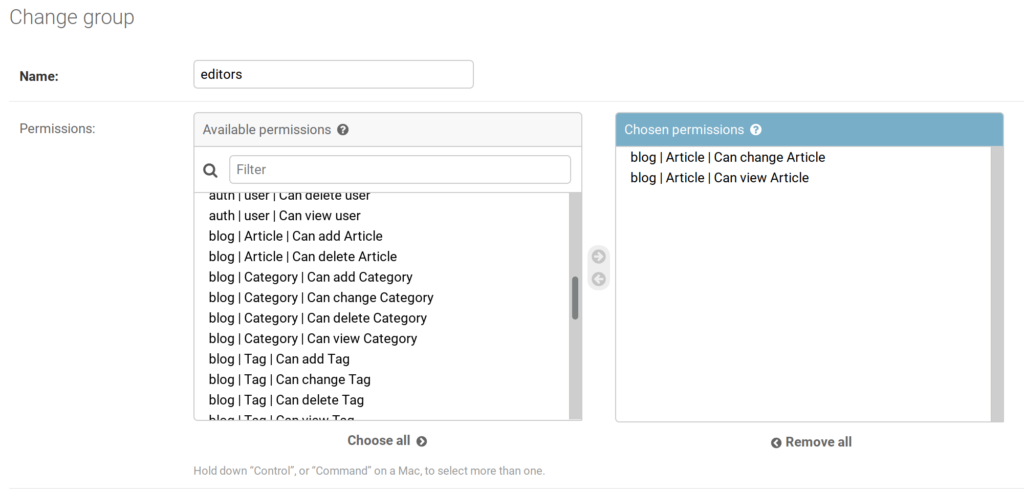
Afterwards we also add the test user to that group (in the user details page). Then when he checks the admin he can see and edit the articles as desired, but not add or delete them.
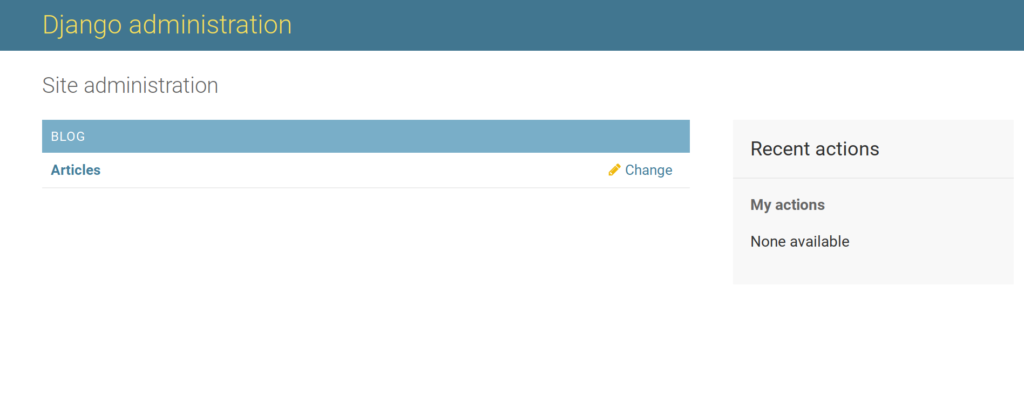
The actions
Down the line, the test user starts doing other kinds of tasks, one of them being “reviewing the orders and then, if everything is correct, mark them as ready for shipment”. In this case, we don’t want him to be able to edit the order details or change anything else, so the existing “update” permissions cannot be used.
What we need now is to create a custom admin action and a new permission that would let specific users (or groups) execute that action. Lets start with the later:
class Order(models.Model):
...
class Meta:
...
permissions = [("set_order_ready", "Can mark the order as ready for shipment")]
What we are doing above, is telling Django there is one more permission that should be created for this model, a permission that we will use ourselves.
Once this is done (you need to run manage.py migrate
), we can now create the action and ensure we check that the user executing it has the newly created permission:
class OrderAdmin(admin.ModelAdmin):
...
actions = ["mark_as_ready"]
def mark_as_ready(self, request, queryset):
if request.user.has_perm("shop.set_order_ready"):
queryset.update(ready=True)
self.message_user(
request, "Selected orders marked as ready", messages.SUCCESS
)
else:
self.message_user(
request, "You are not allowed to execute this action", messages.ERROR
)
mark_as_ready.short_description = "Mark selected orders as ready"
As you can see, we first check the user as the right permission, using has_perm
and the newly defined permission name before proceeding with the changes.
And boom .. now we have this new feature that only lets certain users mark the orders as ready for shipment. If we try to execute this action with the test
user (that does not have yet the required permission):
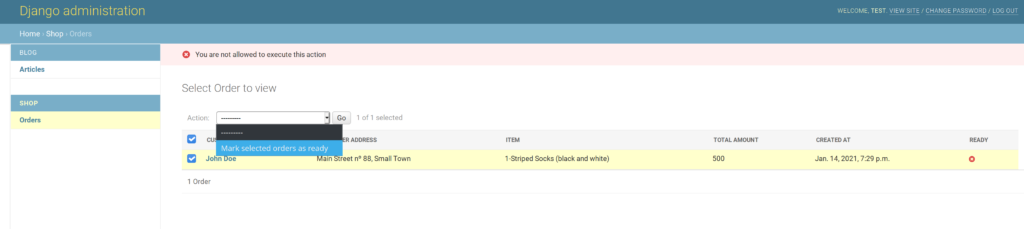
Finally we just add the permission to the user and it’s done. For today this is it, I hope you find it useful.